C++23 Characters
C++ is definitely a language that has Lots of Ways to do It – kind of like Perl’s TIMTOWTSAC. UTF-8 is a suitable source encoding for C++ source, so that you can write string literals in UTF-8. Convenient for adding a poop emoji to an error message, or if your application is very local and doesn’t need translations.
A tiny example (which I have not bothered compiling, YMMV):
QString getSheep() {
return QStringLiteral("🐑");
}
There’s a sheep right there in the source code! But now you’re rather dependent on font support for reading the code – a KDE Plasma desktop comes with sufficient fonts for all your farm animals, but maybe a co-worker doesn’t have a sheep-enabled font and can’t read your code (or review that a sheep is a sheep indeed). There’s a universally-readable solution, though, available with C++23.
\N
escapes.
You can write \N{NAME}
in C++23 source and it compiles down to
a single Unicode character, which becomes however many bytes it needs to be.
This is well-described at CppReference,
which then refers you to the Unicode list of character names.
KDE Plasma users can readily install KCharSelect which knows these names already. Type a search term into the box and you get matching Unicode characters. Off in the details box on the right there is a Name field which you can use.
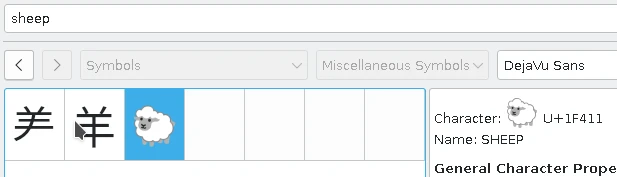
QString getSheep() {
return QStringLiteral("\N{SHEEP}");
}
It might not be quite as pretty, but it is universally readable. Enjoy!
Note that this is not only C++-standard-dependent, but also compiler-dependent. Clang 15 seems to support
\N
regardless of standard, while Clang 13 does not support it at all.